Condition
Introduction
As previously covered in the Conditional execution flow chapter, the Conditional action can be used to control the execution flow by evaluating conditions (or even groups of conditions) at runtime. These conditions are created from the action's settings.
After the conditions have been evaluated, the execution flow will continue it's path through:
- the port with the symbol
0
if the evaluation result isFalse
- the port with the symbol
1
if the evaluation result isTrue
- the error port if there was an error while the conditions were evaluated
Comparison
Let's start with a simple example that compares a user defined variable (let's
assume we've created a variable called foo
and we've assigned bar
as it's
value) with a string.
As mentioned in the Variables chapter,
variable interpolation works in any editable field in the settings of all
actions. Because of this fact, we can compare the value of the variable foo
with any other value or variable.
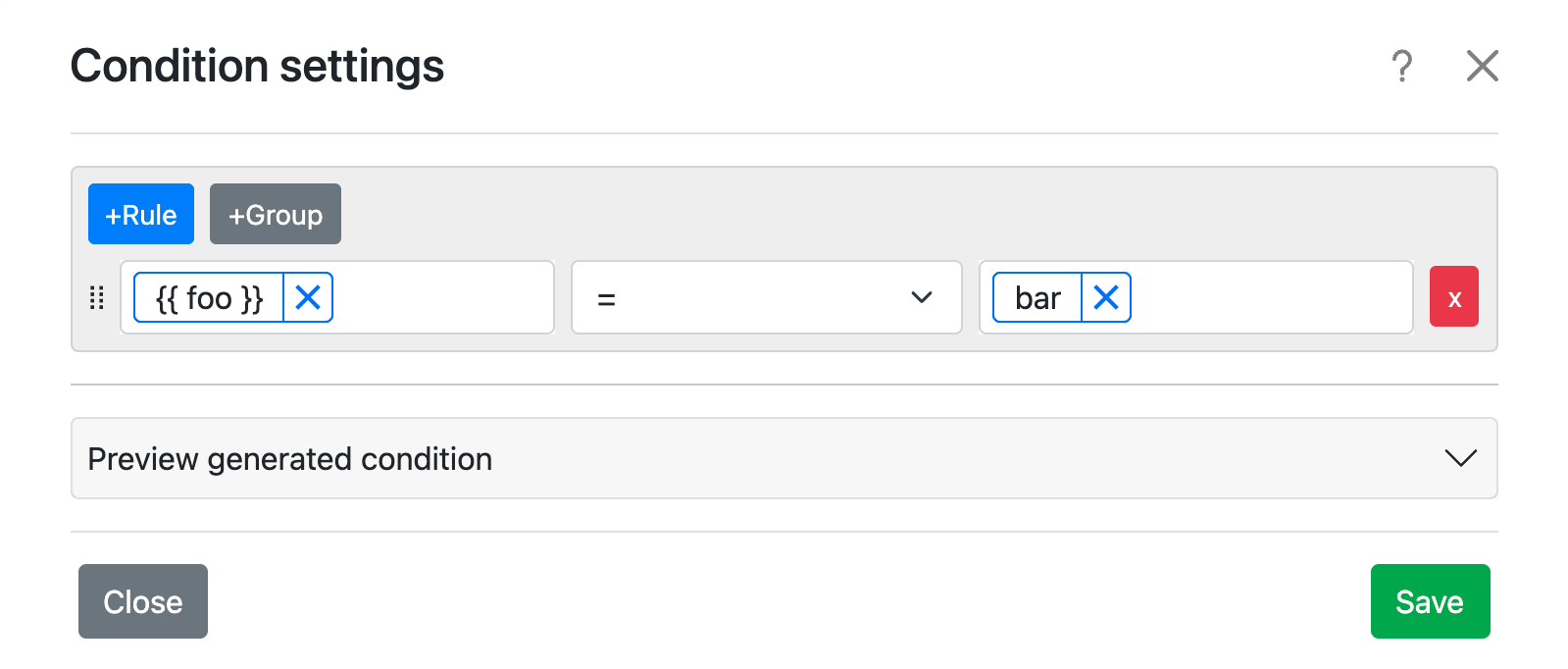
The condition will evaluate to True
, because the value of the variable foo
is equal to bar
.
Following up with a more complex example, let's say that we just ran a script using the Run command action and we want to check the exit value and the output of that script.
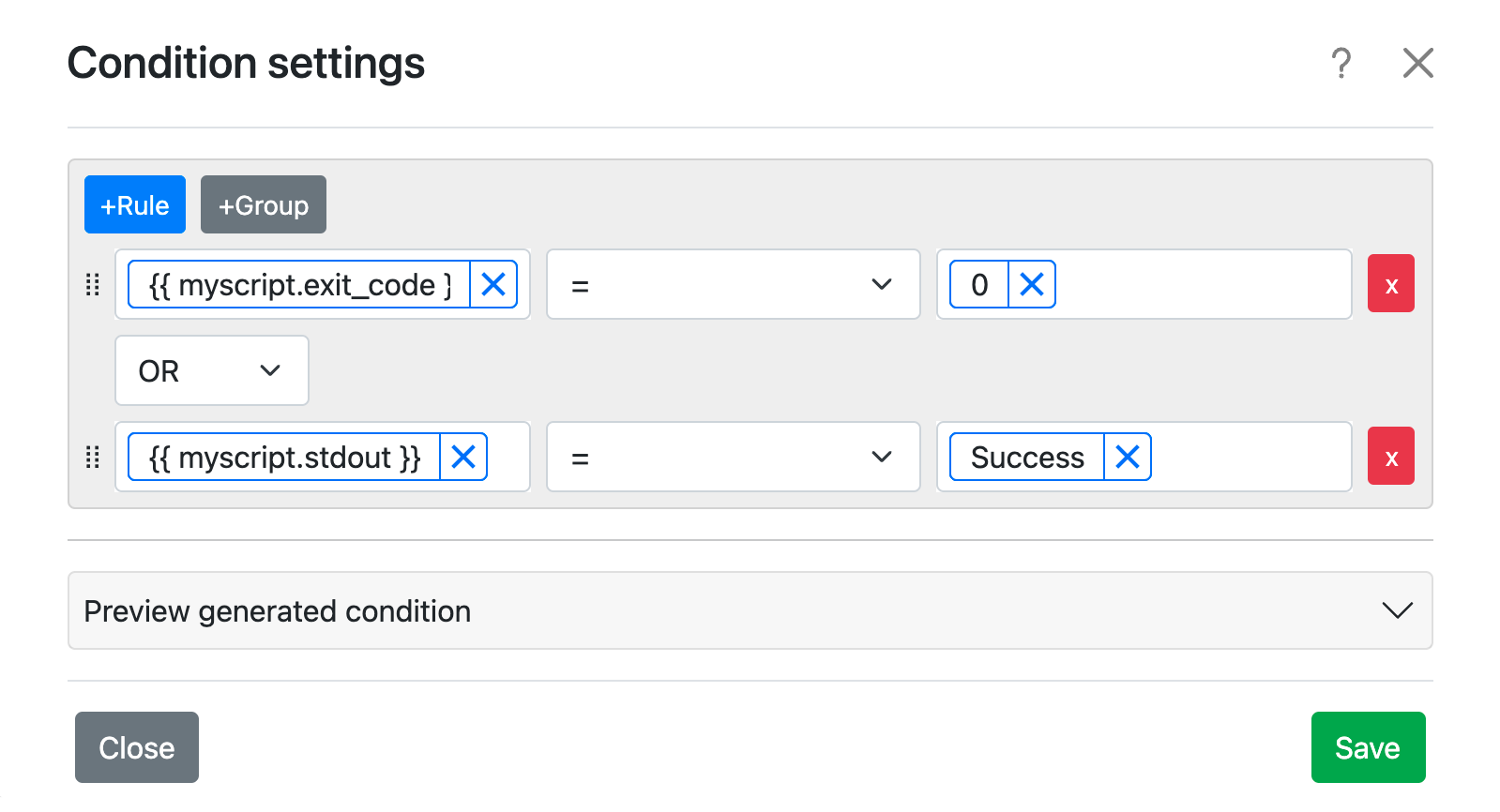
Note that we're comparing the exit_code
, which is an integer, with a 0.
This leads us to the next section of this chapter: Casting.
Casting
Values are not just blindly compared as chunks of raw memory. They are parsed / casted to the type of data they're most likely to fit in, following a specific order.
First of all, each value is tested whether it can be interpreted as an
integer. This is done using
Go's ParseInt, with base 10
and
bitSize 64
. If the value doesn't fit the integer type, float is tried,
using Go's ParseFloat. Then the value
is tested for boolean compatibility using
Go's ParseBool. Finally, if none of the
previous was successful, the value is considered to be a string.
integer -> float -> boolean -> string
Go accepts 1, t, T, TRUE, true, True, 0, f, F, FALSE, false, False
as valid
boolean values.
When a condition is being evaluated, both values must have the same data type. If their types don't match they are casted (using the previously described order of types) until they have the same data type.
Casting examples
Value | Operator | Value | Result after casting | Explanation |
---|---|---|---|---|
1.2 | = | 1 | 1.2 = 1.0 False | 1.2 is not a valid integer, but it's a valid float. Casting stops here. 1 is a valid integer, but it doesn't match the data type of 1.2. It's also a valid float, which does match the data type of 1.2. Casting stops here. |
1.2 | = | true | "1.2" = "true" False | 1.2 is not a valid integer, but it's a valid float. Casting stops here. true is not a valid integer, nor a valid float. It can be casted to a boolean. Casting stops here. Comparison is not possible because of data type mismatch; further casting is required. 1.2 can't be casted to a boolean value, which leaves us with the last data type: string. true must be casted to a string in order to perform comparison. |
false | = | 1 | false = true False | false is not a valid integer, it's not a valid float, but it's a valid boolean value. Casting stops here. 1 is a valid integer. Casting stops here. Comparison is not possible because of data type mismatch; further casting is required. 1 is a valid float, but it doesn't match the data type of false, the next data type to which 1 can be casted is boolean. 1 evaluates to true boolean. |
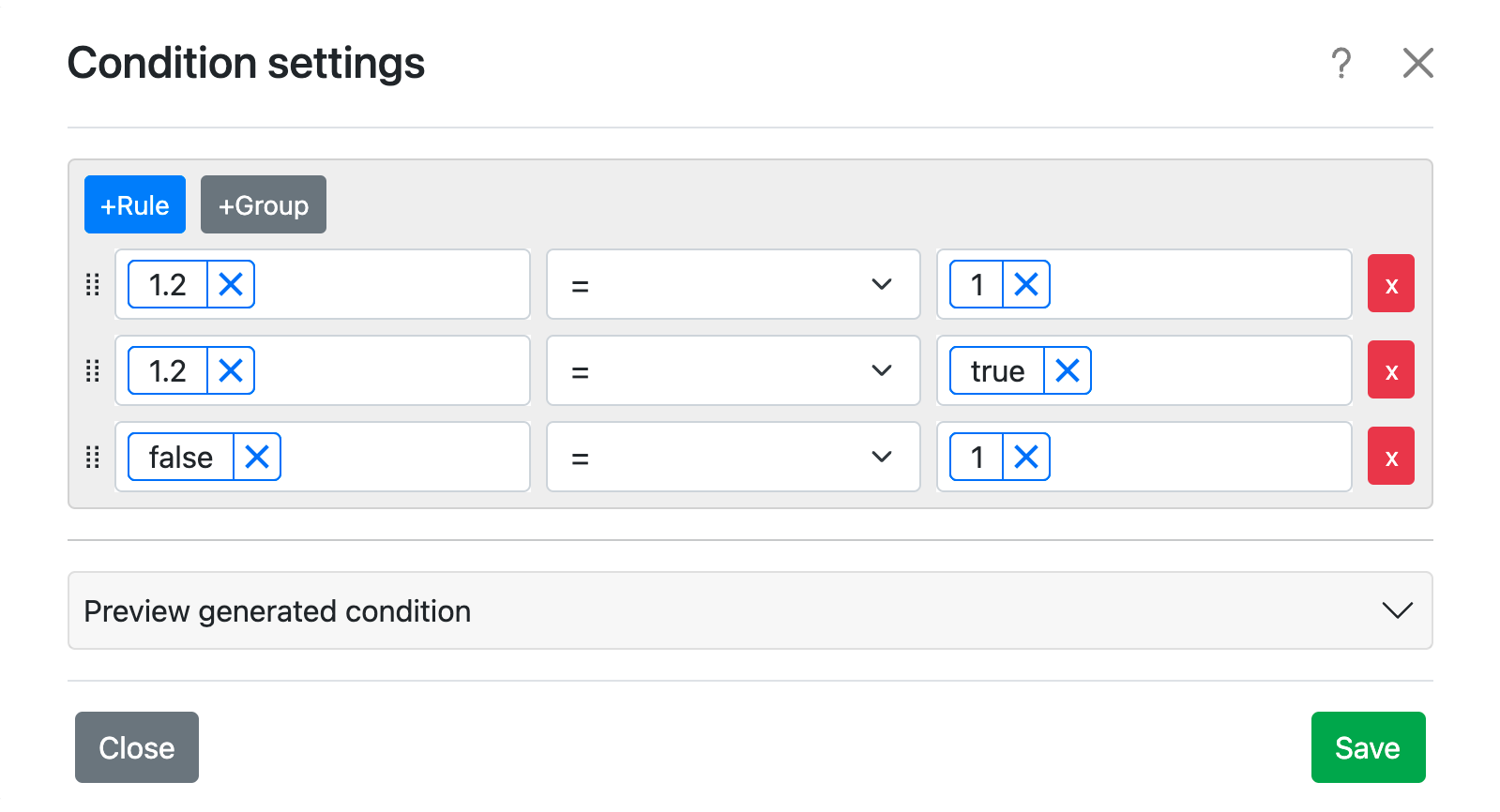
While we do perform casting, we also perform strong type comparison, which means that conditions won't be truthy when comparing a non-empty string and a true boolean, or a positive number and true boolean, etc...
Forcing string type
There might be a case in which you might want to cast a certain value directly as a string. This is totally possible by simple surrounding the value with double quotes.
Errors
We've covered in which occasions the execution flow will continue through the
0
port and in which occasions will do it through the 1
port, but we haven't
covered yet when it might go through the error
port.
Several cases can trigger this behavior:
- error while interpolating any of the variables (inexistent variable or attribute)
- operator not compatible with the data type of the values
Groups
In the event of a very complex condition set, conditions can be grouped. Precedence of logical operators is honored.
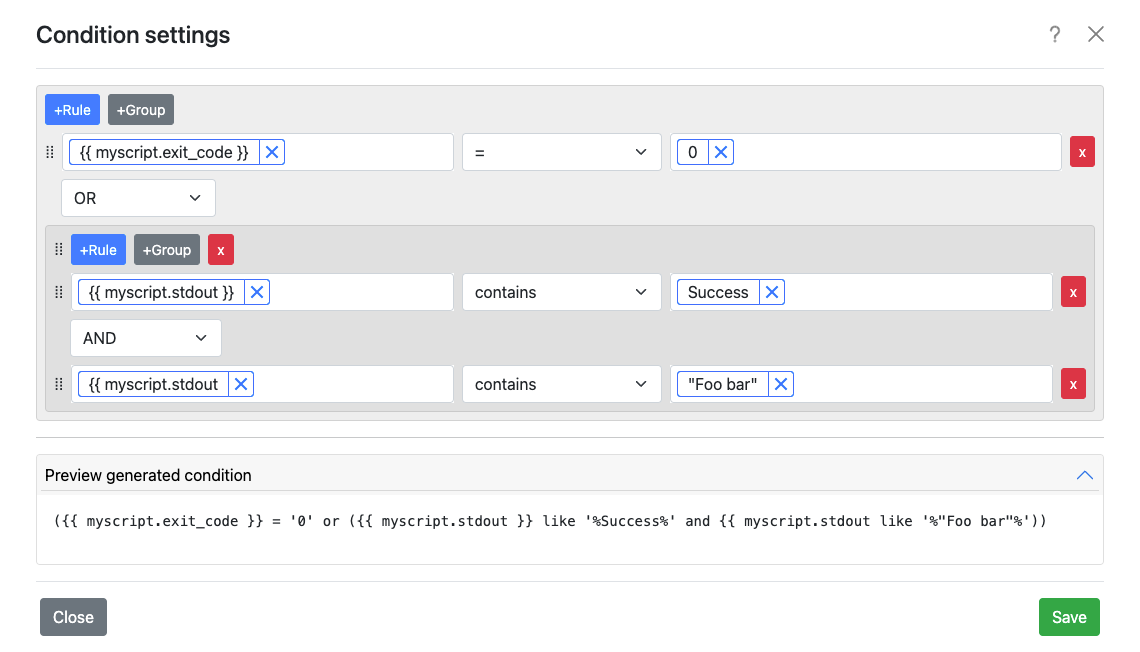
Condition preview
It's possible to preview the conditions using a programming language style syntax.
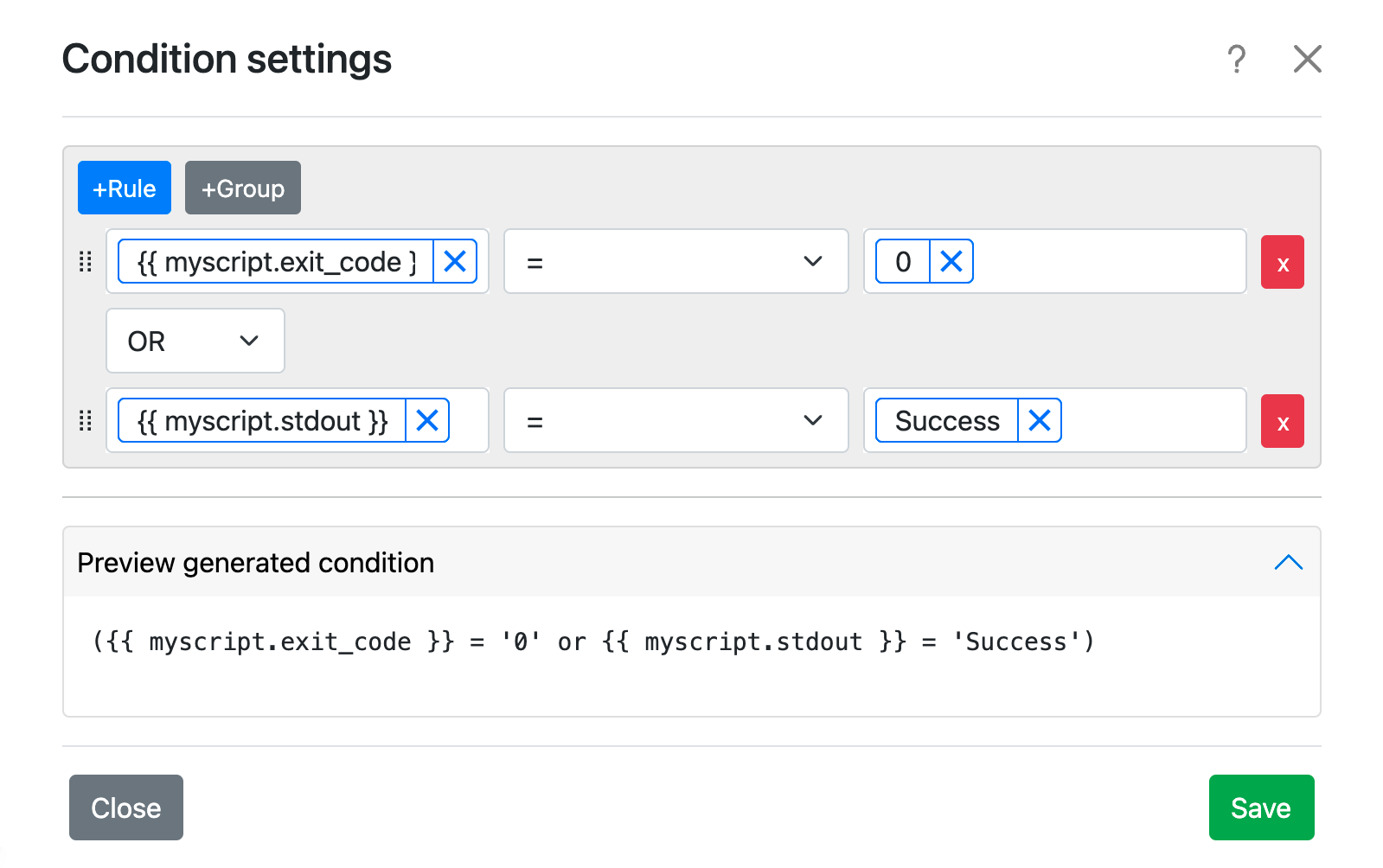